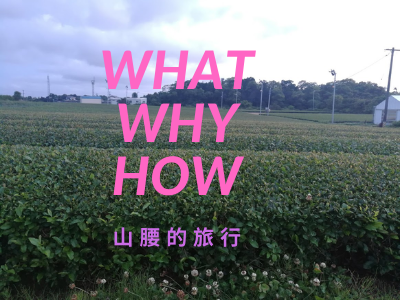
Eigen: environment setup 安装与配置
# Installation
## Install Eigen from source code
```sh
git clone https://gitlab.com/libeigen/eigen.git
```
In fact, the header files in the `Eigen` subdirectory are the only files required to compile programs using Eigen. The header files are the same for all platforms.
- Install using Cmake
```sh
mkdir build
cd build
cmake .. -DCMAKE_INSTALL_PREFIX=myprefix
make
make install
make doc
```
Eigen有用的只是些头文件,由于我一般使用Cmake来搭建自己的工程,所以我还是选择用Cmake编译安装。
这个源码工程本身就是最好的学习资料,推荐使用原码,用原码调试会更方便。
make option:
```sh
---------+--------------------------------------------------------------
Target | Description
---------+--------------------------------------------------------------
install | Install Eigen. Headers will be installed to:
| /
| Using the following values:
| CMAKE_INSTALL_PREFIX: /home/yubao/data/software/eigen
| INCLUDE_INSTALL_DIR: include/eigen3
| Change the install location of Eigen headers using:
| cmake . -DCMAKE_INSTALL_PREFIX=yourprefix
| Or:
| cmake . -DINCLUDE_INSTALL_DIR=yourdir
doc | Generate the API documentation, requires Doxygen & LaTeX
check | Build and run the unit-tests. Read this page:
| http://eigen.tuxfamily.org/index.php?title=Tests
blas | Build BLAS library (not the same thing as Eigen)
uninstall| Remove files installed by the install target
---------+--------------------------------------------------------------
```
## Install Eigen use repo
```sh
sudo apt install libeigen3-dev
```
Where the Eigen is installed:
```sh
sudo updatedb
locate eigen3
```
The default directory is ``/usr/include/eigen3/``
## How to use Eigen in Cmake Project
- Set Eigen3_DIR
如果是使用自己安装的版本的话,需要让Eigen3_DIR指向cmake目录。
Eg.
```sh
export Eigen3_DIR=/home/yubao/data/software/eigen/share/eigen3/cmake
```
- CMakeLists.txt
```sh
cmake_minimum_required (VERSION 3.0)
project (myproject)
find_package (Eigen3 3.3 REQUIRED NO_MODULE)
add_executable (example example.cpp)
target_link_libraries (example Eigen3::Eigen)
```
## How to compile Eigen program without Cmake
```sh
g++ -I /path/to/eigen/ my_program.cpp -o my_program
```
## A simple first program
```sh
#include
#include
using Eigen::MatrixXd;
int main()
{
MatrixXd m(2,2);
m(0,0) = 3;
m(1,0) = 2.5;
m(0,1) = -1;
m(1,1) = m(1,0) + m(0,1);
std::cout << m << std::endl;
}
```
Result:
```sh
3 -1
2.5 1.5
```
# Possible Errors
## Eigen/Core: No such file or directory
```sh
[ 25%] Building CXX object CMakeFiles/useSophus.dir/useSophus.cpp.o
/home/yubao/data/project/slambook2/useSophus/useSophus.cpp:3:10: fatal error: Eigen/Core: No such file or directory
#include
^~~~~~~~~~~~
compilation terminated.
CMakeFiles/useSophus.dir/build.make:62: recipe for target 'CMakeFiles/useSophus.dir/useSophus.cpp.o' failed
make[2]: *** [CMakeFiles/useSophus.dir/useSophus.cpp.o] Error 1
CMakeFiles/Makefile2:67: recipe for target 'CMakeFiles/useSophus.dir/all' failed
make[1]: *** [CMakeFiles/useSophus.dir/all] Error 2
Makefile:83: recipe for target 'all' failed
make: *** [all] Error 2
➜ build git:(master) ✗
```
Solution:
```cpp
#include
```
Refer:
- https://github.com/wjakob/nanogui/issues/356
- ·[fatal error: Eigen/Core: No such file or directory #14868](https://github.com/opencv/opencv/issues/14868)
# References
- [Eigen-Getting started](http://eigen.tuxfamily.org/dox-3.2/GettingStarted.html#title0)
- [Eigen-wiki](http://eigen.tuxfamily.org/index.php?title=Main_Page)
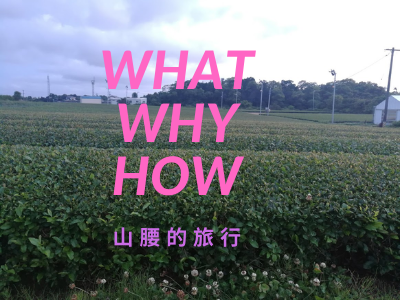
No comments