Python: how to use logging module?
# Objective
- Use logging module
# Example
```python
import logging
# logging.basicConfig(level=logging.DEBUG, format='(%(threadName)-9s) %(message)s',)
# logging.basicConfig(level=logging.INFO)
# logging.basicConfig(filename='example.log',level=logging.DEBUG)
# logging.basicConfig(format='%(asctime)s %(message)s', datefmt='%m/%d/%Y %I:%M:%S %p')
logging.basicConfig(filename='example.log', format='%(asctime)s %(levelname)s:%(message)s', level=logging.DEBUG, datefmt='%m/%d/%Y %I:%M:%S %p')
logging.warning('[warning] Watch out!') # will print a message to the console
logging.info('[info] I told you so') # will not print anything if the level is WARNING
logging.debug('[debug] Starting')
logging.error('[error] Error')
logging.critical('[critical] critical')
logging.warning('%s before you %s', 'Look', 'leap!')
```
Result:
```sh
cat example.log
06/13/2020 02:34:35 PM WARNING:[warning] Watch out!
06/13/2020 02:34:35 PM INFO:[info] I told you so
06/13/2020 02:34:35 PM DEBUG:[debug] Starting
06/13/2020 02:34:35 PM ERROR:[error] Error
06/13/2020 02:34:35 PM CRITICAL:[critical] critical
06/13/2020 02:34:35 PM WARNING:Look before you leap!
```
# Advance example
Refer https://docs.python.org/3/howto/logging.html
```python
import logging
# create logger
logger = logging.getLogger('simple_example')
logger.setLevel(logging.DEBUG)
# create console handler and set level to debug
ch = logging.StreamHandler()
ch.setLevel(logging.DEBUG)
# create formatter
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
# add formatter to ch
ch.setFormatter(formatter)
# add ch to logger
logger.addHandler(ch)
# 'application' code
logger.debug('debug message')
logger.info('info message')
logger.warning('warn message')
logger.error('error message')
logger.critical('critical message')
```
Result:
```sh
python advancedLogging.py
2020-06-13 14:37:59,355 - simple_example - DEBUG - debug message
2020-06-13 14:37:59,355 - simple_example - INFO - info message
2020-06-13 14:37:59,355 - simple_example - WARNING - warn message
2020-06-13 14:37:59,355 - simple_example - ERROR - error message
2020-06-13 14:37:59,355 - simple_example - CRITICAL - critical message
```
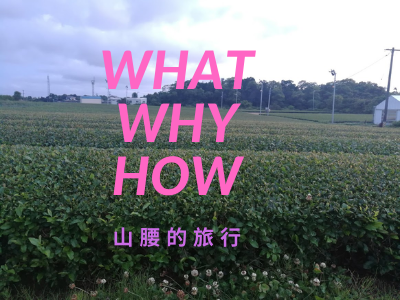
No comments